Topics
Dive into our latest topics to learn, grow, and enhance your JavaScript coding skills.
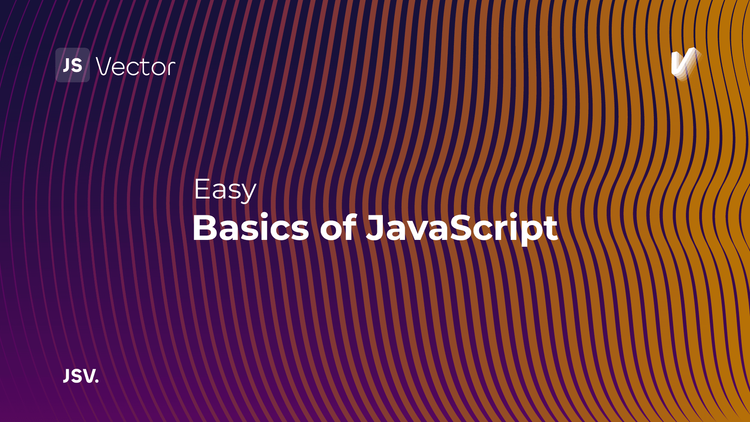
- 1. 1 Introduction to JavaScript: What It Is and Why It Matters
- 1. 2 Setting Up Your JavaScript Development Environment
- 1. 3 Variables in JavaScript: var, let, and const
- 1. 4 Basic Data Types in JavaScript
- 1. 5 Understanding Operators in JavaScript
- 1. 6 Control Structures: If Statements and Loops in JavaScript
- 1. 7 Functions in JavaScript: Defining and Invoking
- 1. 8 The Document Object Model (DOM): An Introduction
- 1. 9 Handling Events in JavaScript
- 1. 10 Forms and Validation with JavaScript
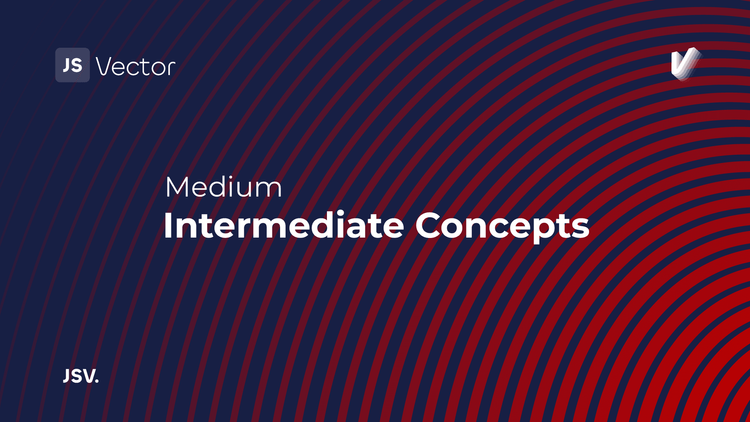
- 2. 1 Arrays in JavaScript: Operations and Methods
- 2. 2 JavaScript Objects and Prototypes
- 2. 3 Understanding Scope and Closure in JavaScript
- 2. 4 Error Handling and Debugging in JavaScript
- 2. 5 Introduction to Let, Const and Arrow Functions
- 2. 6 Template Literals and Enhanced Object Literals in JavaScript
- 2. 7 Destructuring Assignments in JavaScript
- 2. 8 Spread and Rest Operators in JavaScript
- 2. 9 Iterators and Generators in JavaScript
- 2. 10 Modules in JavaScript: Import and Export
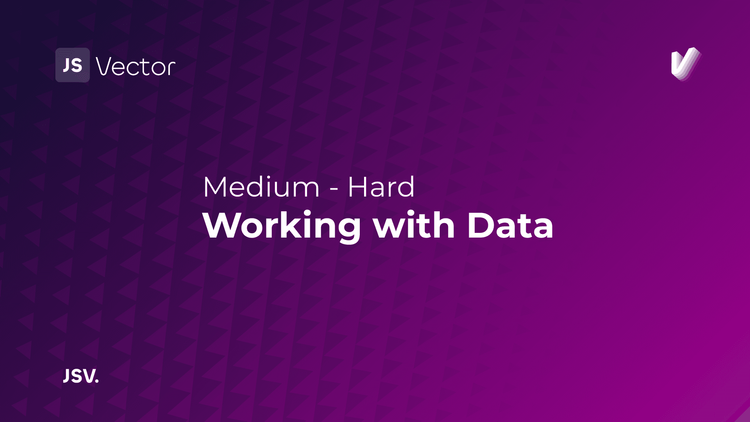
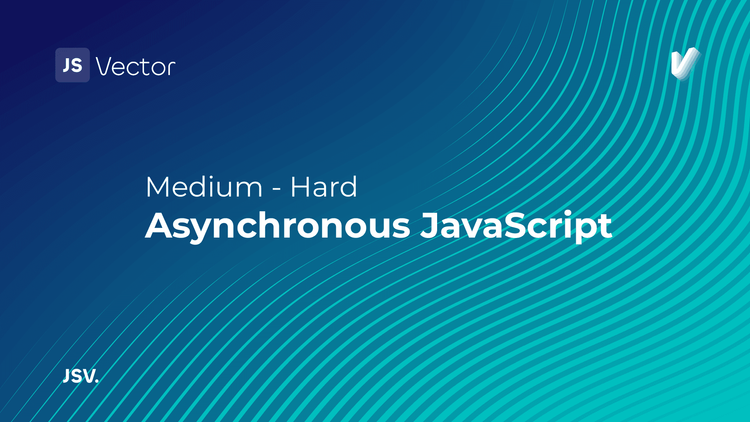
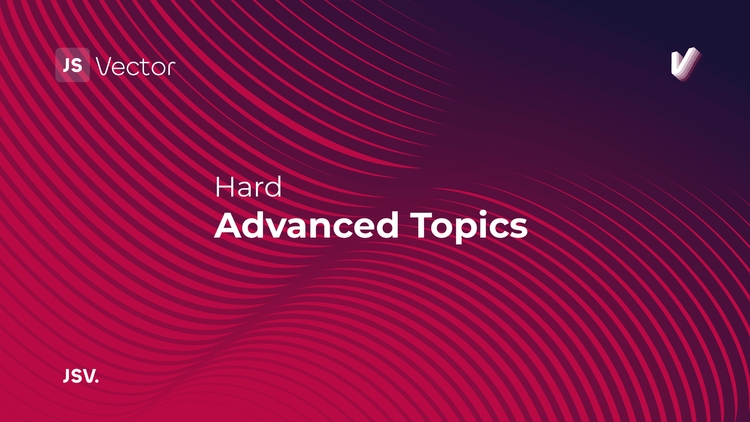
- 5. 1 Deep Dive into JavaScript Closures
- 5. 2 Understanding 'this' Keyword in Depth
- 5. 3 Prototype Inheritance and Chain in JavaScript
- 5. 4 Event Loops and JavaScript Runtime
- 5. 5 Memory Management in JavaScript
- 5. 6 JavaScript Design Patterns
- 5. 7 Writing Clean Code with JavaScript
- 5. 8 Best Security Practices in JavaScript
- 5. 9 Performance Optimization in JavaScript
- 5. 10 Testing JavaScript Code: Unit Tests and Integration Tests
- 5. 11 Introduction to Service Workers and Progressive Web Apps
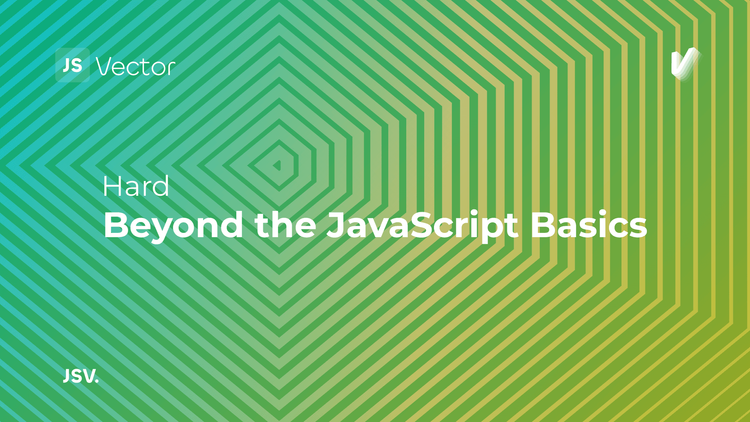
- 6. 1 Building a Simple Web Application with Vanilla JavaScript
- 6. 2 Creating Interactive Web Pages with JavaScript
- 6. 3 Introduction to Canvas API for Graphics
- 6. 4 Introduction to Web Audio API
- 6. 5 Using the Geolocation API in JavaScript
- 6. 6 Building Offline-First Web Applications with JavaScript
- 6. 7 Introduction to WebAssembly and its Usage with JavaScript
- 6. 8 Creating Extensions for Web Browsers
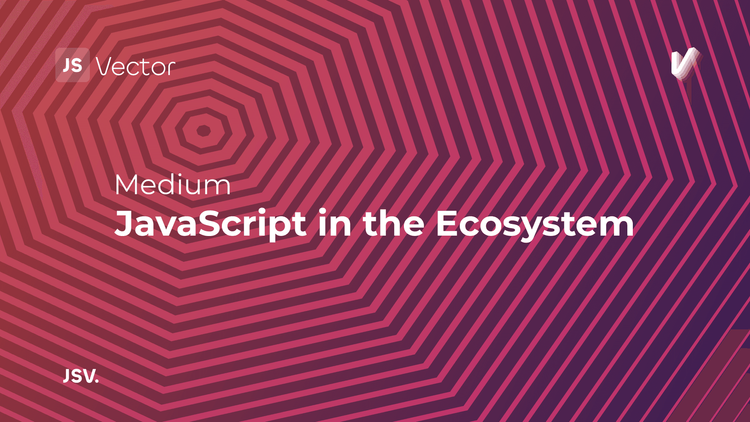
- 7. 1 Integrating JavaScript with HTML and CSS for Web Development
- 7. 2 Using JavaScript to Manipulate CSS Styles
- 7. 3 Build a Basic SPA (Single Page Application) with JavaScript
- 7. 4 Introduction to SEO and JavaScript
- 7. 5 JavaScript and Accessibility: Best Practices
- 7. 6 Internationalization and Localization with JavaScript
- 7. 7 Server-Side JavaScript with Node.js: An Introduction
- 7. 8 Building RESTful APIs with Node.js and Express
- 7. 9 Real-Time Applications with Socket.IO
- 7. 10 Static Site Generation with JavaScript